to a target site or site collection through a process known as feature activation. The element types that can be defined by a feature include menu commands, link commands, page templates, page instances, list definitions, list instances, event handlers, and workflows.
At a physical level, a feature consists of a directory created within a special WSS system directory located within the file system of each front-end Web server. The directory for a feature contains one or more XML-based files that contain Collaborative Application Markup Language (CAML). By convention, each feature directory contains a manifest file named feature.xml that defines the high-level attributes of the feature, such as its ID and its userfriendly Title.
Programming Against the WSS Object Model
static void Main() {
string sitePath = "http://litwareinc.com";
// enter object model through site collection.
SPSite siteCollection = new SPSite(sitePath);
// obtain reference to top-level site.
SPWeb site = siteCollection.RootWeb;
// enumerate through lists of site
foreach (SPList list in site.Lists) {
if(!list.Hidden)
Console.WriteLine(list.Title);
}
// clean up by calling Dispose.
site.Dispose();
siteCollection.Dispose();
}
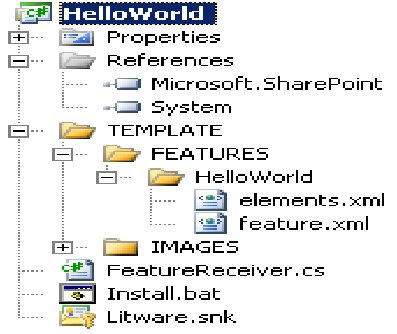
Future.xml
feature id="B2CB42E2-4F0A-4380-AABA-1EF9CD526F20" title="A Sample Feature: Hello World" xmlns="http://schemas.microsoft.com/sharepoint/" description="This demoware was created by NaveedShare-LicenseToPlay " scope="Web" hidden="FALSE" imageurl="TPG\WhitePithHelmet.gif" receiverassembly="HelloWorld, Version=1.0.0.0, Culture=neutral, PublicKeyToken=b38a04419cc857d9" receiverclass="HelloWorld.FeatureReceiver">
elementmanifests>
elementmanifest location="elements.xml">
/elementmanifests>
/feature>
elements.xml
elements xmlns="http://schemas.microsoft.com/sharepoint/">
customaction id="SiteActionsToolbar" title="Hello World" description="A custom menu item added using a feature" imageurl="_layouts/images/crtsite.gif" location="Microsoft.SharePoint.StandardMenu" groupid="SiteActions" sequence="100">
urlaction url="http://msdn.microsoft.com">
/customaction>
/elements>
FeatureReceiver.cs
using System;
using Microsoft.SharePoint;
namespace HelloWorld {
public class FeatureReceiver : SPFeatureReceiver {
// no functionality required for install/uninstall events
public override void FeatureInstalled(SPFeatureReceiverProperties properties) { }
public override void FeatureUninstalling(SPFeatureReceiverProperties properties) { }
public override void FeatureActivated(SPFeatureReceiverProperties properties) {
SPWeb site = (SPWeb)properties.Feature.Parent;
// track original site Title using SPWeb property bag
site.Properties["OriginalTitle"] = site.Title;
site.Properties.Update();
// update site title
site.Title = "Hello World";
site.Update();
}
public override void FeatureDeactivating(SPFeatureReceiverProperties properties) {
// reset site Title back to its original value
SPWeb site = (SPWeb)properties.Feature.Parent;
site.Title = site.Properties["OriginalTitle"];
site.Update();
}
}
}
Install.bat
@SET TEMPLATEDIR="c:\program files\common files\microsoft shared\web server extensions\12\Template"
@SET STSADM="c:\program files\common files\microsoft shared\web server extensions\12\bin\stsadm"
@SET GACUTIL="c:\Program Files\Microsoft Visual Studio 8\SDK\v2.0\Bin\gacutil.exe"
Echo Installing HelloWorld.dll in GAC
%GACUTIL% -if bin\debug\HelloWorld.dll
Echo Copying files to TEMPLATE directory
xcopy /e /y TEMPLATE\* %TEMPLATEDIR%
Echo Installing feature
%STSADM% -o installfeature -filename HelloWorld\feature.xml -force
IISRESET
REM cscript c:\windows\system32\iisapp.vbs /a "SharePointDefaultAppPool" /r
Once you have added the install.bat file, you can configure Visual Studio to run it each time you rebuild the HelloWorld project by going to the Build Events tab within the Project Properties and adding the following post-build event command line instructions.
cd $(ProjectDir)
Install.bat
The properties parameter is based on the SPFeatureReceiverProperties class that exposes a Feature property that, in turn, exposes a Parent property that holds a reference to the current site.
No comments:
Post a Comment